Intro
Introduction to Data Structures & Algorithms
Understanding data structures is crucial for efficient problem-solving in computer science. This guide offers a comprehensive exploration of key data structures, including arrays, linked lists, stacks, queues, trees, and graphs. Each structure is presented with clear explanations, real-world applications, and practical examples to help you master the fundamentals and enhance your coding skills. Dive into the core concepts and unlock the power of data structures for optimized algorithm design and software development.
Data Structures and Algorithms:
Let's clear up our basics with these terms before deep diving into DSA. Data Structures and Algorithms are two different things.
Data Structures – Data structures are the building blocks for constructing efficient algorithms. It is like how tools can be used to help you organize data in ways that will be much easier and quicker to use from the computer's memory. Examples of common data structures include arrays, stacks, and linked lists. Don't worry too much about the names, but just be aware that they'll help you access and manipulate your data in a more efficient way.
Algorithms – An algorithm is a series of steps on data performed to solve a problem, and the efficient use of data structures helps make the process faster and easier. So whether one's problem is simple or complex, like sorting an array, the right use of data structure will ensure that the best method for handling the data will be adopted while saving much time and resources.
Some other Important terminologies:
-
Database – Data that would be saved for quick retrieval and later updates would be stored in a repository of permanent data storage. It hence guarantees the availability of data any time it is needed. Some databases, for instance are MySQL and MongoDB, storing data in a selected order for easy access and modification.
-
Data warehouse – Management of large legacy data has to maintain older data differently from current or fresh data in a database. Legacy data stored outside the latest or current data of a database will increase retrieval time and save precious database time for analysis. So, put legacy data in one location and call for it when required; in this way, legacy data does not clutter up the place and keeps the database running smoothly while fetching better analyses and performance.
-
Big data – In big data analysis, when data is of a size and complexity that cannot be handled by traditional data processing tools, we use specialized methods for analysis. Advanced technologies and algorithms correctly process vast amounts of information in a manner that would otherwise be too overwhelming for regular systems to manage, thereby extracting good insights from data.
Memory Layout of Programs:
When the program starts, its code gets copied to the main memory.
- The stack holds the memory occupied by functions. It stores the activation records of the functions used in the program. And erases them as they get executed.
- The heap contains the data which is requested by the program as dynamic memory using pointers.
- Initialized and uninitialized data segments hold initialized and uninitialized global variables, respectively.
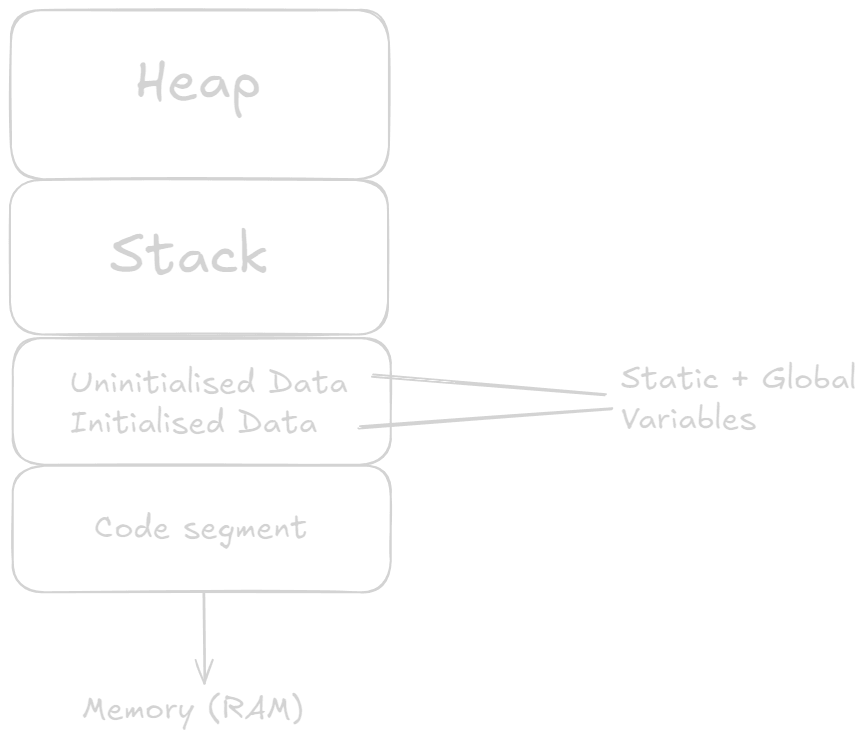
So, this was all for the beginning. Data Structures and Algorithms are not new concepts. If you have done programming in any language like C, you must have come across Arrays – A data structure. And algorithms are just sequences of processing steps to solve a problem. :)